mirror of
https://github.com/foo-dogsquared/wiki.git
synced 2025-01-30 22:57:59 +00:00
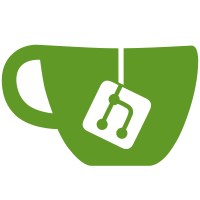
There are different recipes or at least tidbits that are essentially recipes so we'll just put them under separate namespaces in the hierarchy.
3.2 KiB
3.2 KiB
Code recipes: Implementing tiered headings with CSS
You may have seen certain documents with tiered headings especially in technical manuals and specifications (e.g., ECMAScript, HTML). To implement this with pure CSS, we'll make heavy use of CSS counters.
Here is one rough implementation with SCSS.
article {
// Named after the LaTeX counters after `chapter` counter.
counter-reset: section subsection subsubsection paragraph subparagraph;
--counter-spacing: 0.5rem;
// <h2> is used as a section header since <h1> is the main title
h2 {
counter-reset: subsection;
counter-increment: section;
&::before {
content: counter(section) ".";
margin-right: var(--counter-spacing);
}
}
h3 {
counter-reset: subsubsection;
counter-increment: subsection;
&::before {
content: counter(section) "." counter(subsection) ".";
margin-right: var(--counter-spacing);
}
}
h4 {
counter-reset: paragraph;
counter-increment: subsubsection;
&::before {
content: counter(section) "." counter(subsection) "." counter(subsubsection) ".";
margin-right: var(--counter-spacing);
}
}
h5 {
counter-reset: subparagraph;
counter-increment: paragraph;
&::before {
content: counter(section) "." counter(subsection) "." counter(subsubsection) "." counter(paragraph) ".";
margin-right: var(--counter-spacing);
}
}
h6 {
counter-increment: subparagraph;
&::before {
content: counter(section) "." counter(subsection) "." counter(subsubsection) "." counter(paragraph) "." counter(subparagraph);
margin-right: var(--counter-spacing);
}
}
}
This is enough for CSS but since we're using SCSS, we can optimize it further. You may notice that each level is similar with the difference being the content and their counter name.
@use 'sass:string';
@function tier-heading($counters) {
$str: "";
@for $level from 1 through $counters {
$str: $str + 'counter(h#{$level})"."';
}
@return string.unquote($str);
}
article {
--counter-spacing: 0.5rem;
@for $level from 1 through 6 {
counter-reset: h#{$level};
h#{$level}{
@if $level != 6 {
counter-reset: h#{$level + 1};
}
counter-increment: h#{$level};
&::before {
content: tier-heading($level);
margin-right: var(--counter-spacing);
}
}
}
}